必须要了解的Python关键词
2020-06-23
作者:老齐
与本文相关图书推荐:《Python大学实用教程》
本书适合于零基础的初学者,书中详细介绍了Python语言,特别是配有相关练习题。
每种编程语言都会有一些特殊的单词,称为关键词。对待关键词的基本要求是,你在命名的时候要避免与之重复。本文将介绍一下Python中的关键词。关键词不是内置函数或者内置对象类型,虽然在命名的时候同样也最好不要与这些重名,但是,毕竟你还可以使用与内置函数或者内置对象类型重名的名称来命名。关键词则不同,它是不允许你使用。
在Python3.8中提供了35个关键词,如下所示:
1 | False await else import pass |
你可以通过下面的链接,查看官方文档对每个关键词的说明:https://docs.python.org/3.8/reference/lexical_analysis.html#keywords
如果打算在交互模式里面查看关键词,可以使用help()
:
1 | "keywords") help( |
对每个关键词的详细说明,也可以用help()
查看:
1 | 'pass') # 敲回车后出现下面的内容 help( |
除了上面的方法之外,还有一个标准库的模块keyword
提供了关键词查询功能。
1 | import keyword |
那么,这些关键词如何使用?在什么情景下应用?下面以示例的方式对部分关键词进行说明。
True、False和None
True
和False
是布尔类型的两个值,注意必须首字母大写。
1 | True x = |
如果我们要判断某个对象的布尔值是True
还是False
,可以使用bool()
函数实现,例如:
1 | "this is a truthy value" x = |
注意,如果向bool()
传入的参数是0, "", {}, []
中的任何一个,返回值都是False
。
在条件语句中,本来是要判断条件是否为True
,但是,通常不需要直接与True
或者False
进行比较,依靠Python解析器自动进行条件判断。
1 | "this is a truthy value" x = |
None
这个关键词,在Python中表示没有值,其他语言中,同样的含义可能会用null,nil,none,undef,undefined
等。None
也是函数中没有return
语句的时候默认返回值。
1 | def func(): |
and、or、not、in、is
这几个关键词,其实都对应着数学中的操作符,如下表所示。
数学符号 | 关键词 |
---|---|
AND, ∧ | and |
OR, ∨ | or |
NOT, ¬ | not |
CONTAINS, ∈ | in |
IDENTITY | is |
Python代码具有很强的可读性,通过关键词就能一目了然晓得是什么操作。
这几个关键词比较好理解,这里仅提醒注意在Python中有一个著名的短路运算,例如and
:
1 | <expr1> and <expr2> |
不要将上面的式子理解成两边都是真的时候返回True
。对此,在《Python大学实用教程》一书中有非常详细的说明,请参阅。另外一个就是or
,也存在短路运算。
break、continue和else
这几个是经常用于循环语句的关键词。
break
的作用是终止当前循环,其使用的基本格式:
1 | for <element> in <container>: |
举个例子:
1 | 1, 2, 3, 4, 5, 6, 7, 8, 9, 10] nums = [ |
continue
则是要跳过某些循环,然后让循环继续。
1 | for <element> in <container>: |
else
在条件语句中有,这里提到它,是在循环语句中,它的作用是当循环结束后还要继续执行的代码。
在for循环中,使用格式如下:
1 | for <element> in <container>: |
在while循环中,使用格式如下:
1 | while <expr>: |
例如,有时候我们要在循环语句中使用一个旗帜变量:
1 | for n in range(2, 10): |
在上面的代码中,prime
就是一个旗帜变量,如果循环正常结束,prime
的值就是True
,否则,就是False
。如果从循环中退出了,第8行判断这个变量的值,如果为True
则打印相应内容。
对于上面的代码,如果用else
改写,可以更简洁,并且可读性更强。
1 | >>> for n in range(2, 10): |
其他一些关键词,本文不再赘述,可以参考《Python大学实用教程》中的有关内容。
若你觉得我的文章对你有帮助,欢迎点击上方按钮对我打赏
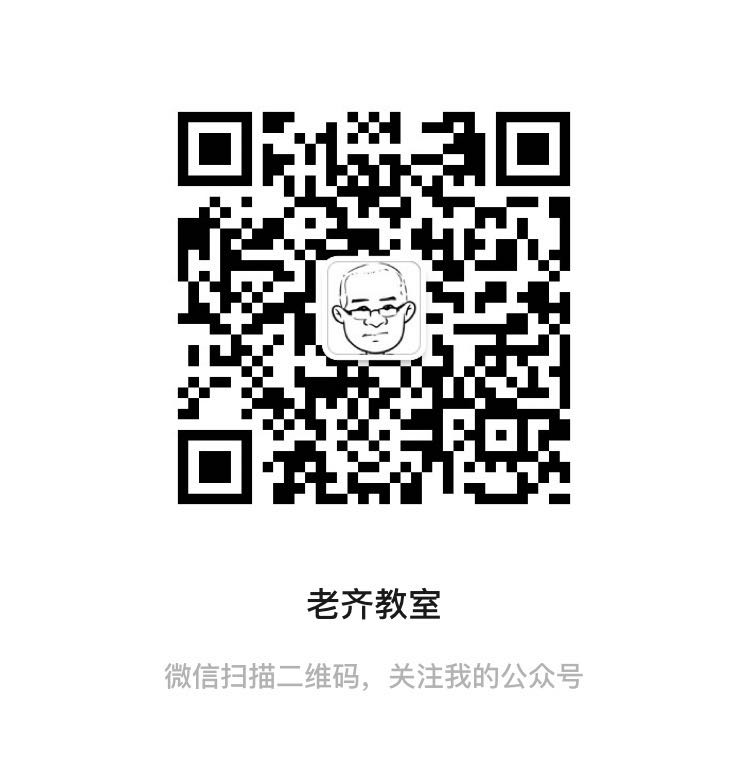
关注微信公众号,读文章、听课程,提升技能